Internal Workings of Go, Python, Rust, and C#
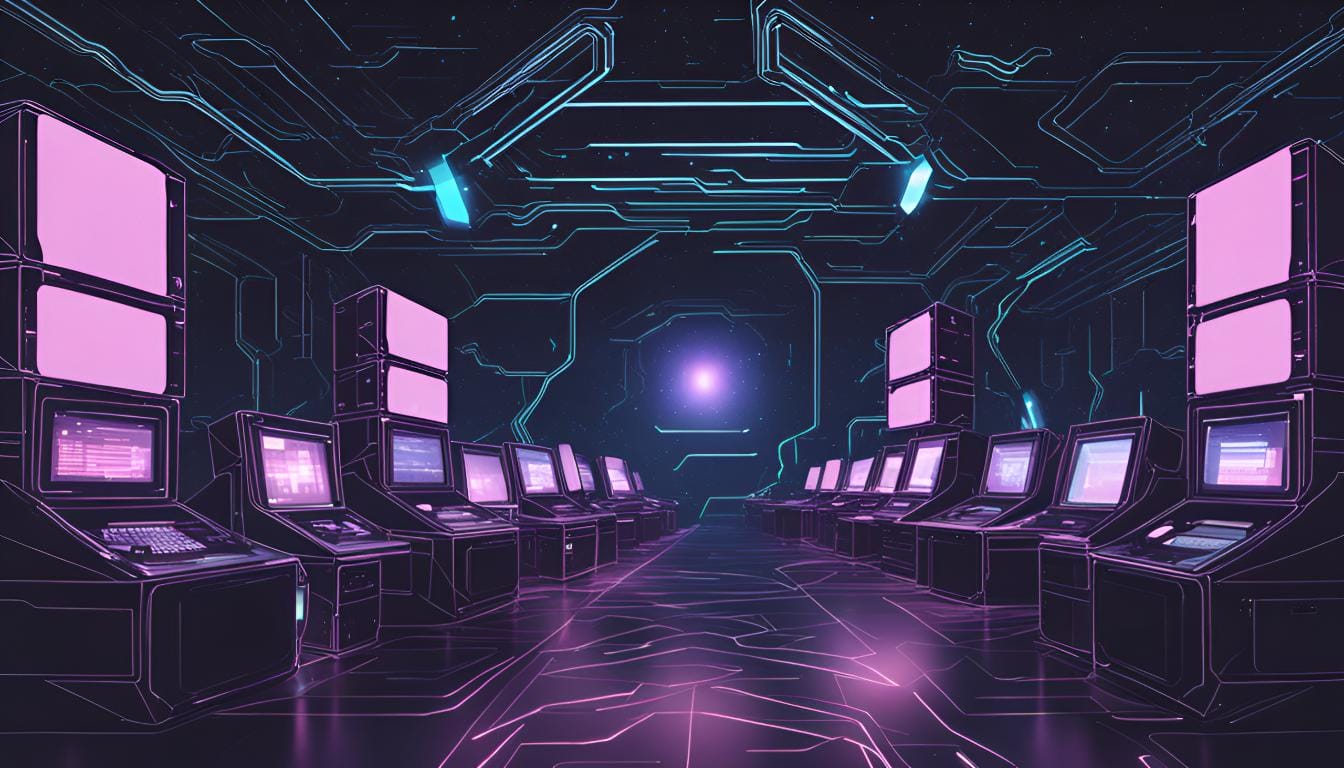
Go (Golang)
Compilation and Execution
Go uses a compile-time approach, where source code is translated directly into machine code. The compilation process involves several stages:
- Lexical analysis
- Syntax parsing
- Type checking and AST generation
- Intermediate code generation
- Machine code generation and optimization
The resulting binary is platform-specific and can be executed directly by the operating system.
Memory Management
Go employs a concurrent mark-and-sweep garbage collector. Key features include:
- Tri-color marking algorithm
- Write barriers for concurrent marking
- Parallel marking and sweeping phases
Go's memory allocator uses a segregated-fit algorithm with per-size classes and per-thread caches to reduce lock contention.
Concurrency Model
Go's concurrency is built around goroutines and channels:
- Goroutines are lightweight threads managed by the Go runtime
- The runtime multiplexes goroutines onto OS threads using a work-stealing scheduler
- Channels provide a way for goroutines to communicate and synchronize
Python
Execution Model
Python uses an interpreter-based approach:
- Source code is compiled to bytecode
- Bytecode is executed by the Python Virtual Machine (PVM)
The PVM is a stack-based machine that executes bytecode instructions sequentially.
Memory Management
Python's memory management involves:
- Reference counting as the primary mechanism
- Generational garbage collection to handle circular references
- Memory allocation using a custom allocator (PyMalloc) for small objects
The interpreter maintains a private heap containing all Python objects and data structures.
Global Interpreter Lock (GIL)
The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecode at once. While it simplifies the implementation of the interpreter, it can limit performance in CPU-bound and multi-threaded code.
Rust
Compilation Process
Rust's compilation involves several stages:
- Parsing the source code into an Abstract Syntax Tree (AST)
- Lowering the AST to High-Level Intermediate Representation (HIR)
- Type checking and borrow checking
- Translation to Mid-level Intermediate Representation (MIR)
- Optimization passes on MIR
- Code generation using LLVM
Memory Management
Rust's unique approach to memory management is based on ownership rules:
- Each value has a single owner
- When the owner goes out of scope, the value is dropped
- Ownership can be transferred (moved) or borrowed
The borrow checker enforces these rules at compile-time, preventing common memory-related errors without runtime
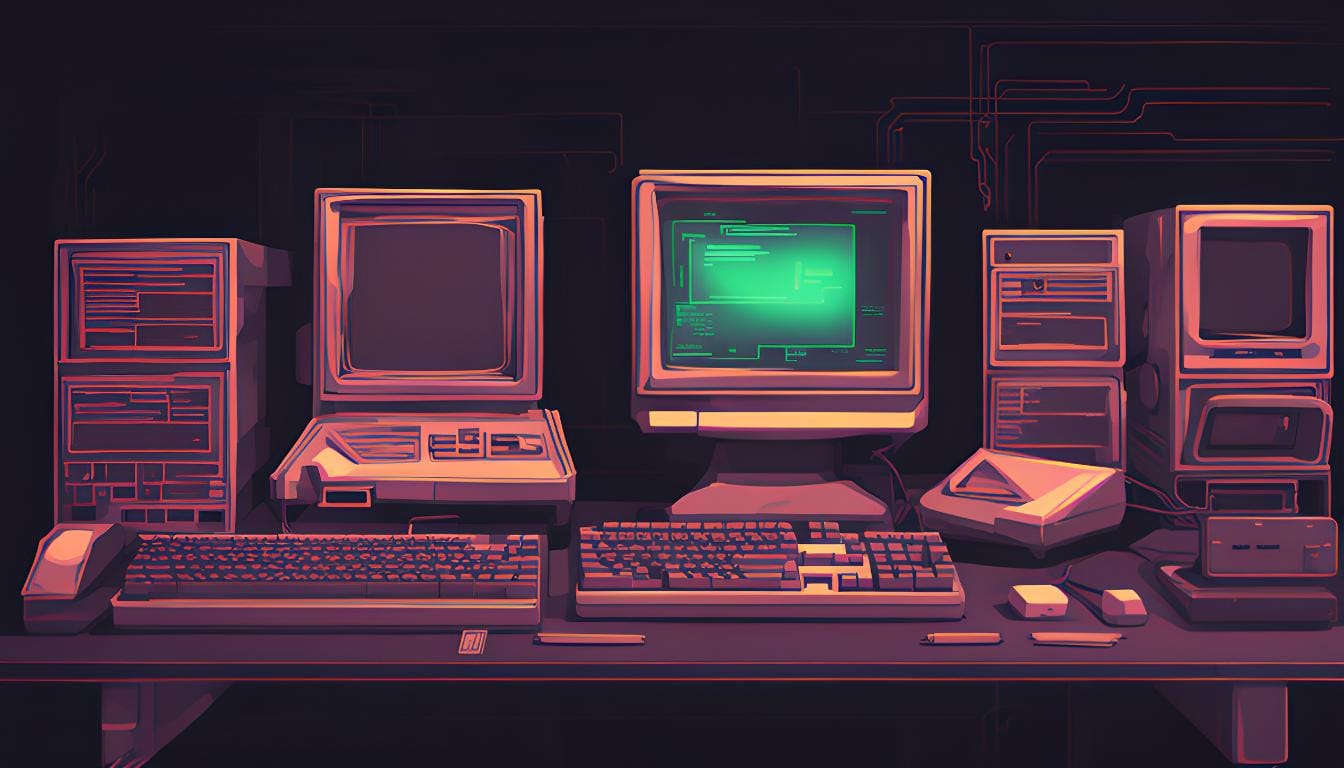
Code Examples and Low-Level Analysis
Go
Let's examine a simple Go program and its interaction with memory:
package main
import "fmt"
func main() {
a := 100
b := 21
c := add(a, b)
fmt.Printf("The result is: %d\n", c)
}
func add(x, y int) int {
return x + y
}
At the assembly level (using go tool compile -S
), we can see how Go manages the stack and performs the addition:
"".main STEXT size=128 args=0x0 locals=0x40
// Function prologue
0x0000 00000 (example.go:5) TEXT "".main(SB), ABIInternal, $64-0
0x0000 00000 (example.go:5) MOVQ (TLS), CX
0x0009 00009 (example.go:5) CMPQ SP, 16(CX)
0x000d 00013 (example.go:5) PCDATA $0, $-2
0x000d 00013 (example.go:5) JLS 118
0x000f 00015 (example.go:5) PCDATA $0, $-1
0x000f 00015 (example.go:5) SUBQ $64, SP
0x0013 00019 (example.go:5) MOVQ BP, 56(SP)
0x0018 00024 (example.go:5) LEAQ 56(SP), BP
// Main function body
0x001d 00029 (example.go:6) MOVQ $100, "".a+40(SP)
0x0026 00038 (example.go:7) MOVQ $21, "".b+32(SP)
0x002f 00047 (example.go:8) MOVQ "".a+40(SP), AX
0x0034 00052 (example.go:8) MOVQ "".b+32(SP), BX
0x0039 00057 (example.go:8) CALL "".add(SB)
0x003e 00062 (example.go:8) MOVQ AX, "".c+24(SP)
// ... (printf call and function epilogue)
This assembly code shows how Go manages the stack frame, allocates local variables, and performs the function call.
Python
In Python, we can use the dis
module to examine the bytecode:
import dis
def add(x, y):
return x + y
def main():
a = 100
b = 21
c = add(a, b)
print(f"The result is: {c}")
dis.dis(main)
Output:
5 0 LOAD_CONST 1 (100)
2 STORE_FAST 0 (a)
6 4 LOAD_CONST 2 (21)
6 STORE_FAST 1 (b)
7 8 LOAD_GLOBAL 0 (add)
10 LOAD_FAST 0 (a)
12 LOAD_FAST 1 (b)
14 CALL_FUNCTION 2
16 STORE_FAST 2 (c)
8 18 LOAD_GLOBAL 1 (print)
20 LOAD_CONST 3 ('The result is: ')
22 LOAD_FAST 2 (c)
24 FORMAT_VALUE 0
26 BUILD_STRING 2
28 CALL_FUNCTION 1
30 POP_TOP
32 LOAD_CONST 0 (None)
34 RETURN_VALUE
This bytecode shows how Python loads constants, stores variables, and performs function calls using its stack-based virtual machine.
Rust
In Rust, we can use the --emit asm
flag to see the assembly output:
fn add(x: i32, y: i32) -> i32 {
x + y
}
fn main() {
let a = 100;
let b = 21;
let c = add(a, b);
println!("The result is: {}", c);
}
Compiled with rustc -C opt-level=0 --emit asm example.rs
, we get:
example::add:
push rbp
mov rbp, rsp
mov dword ptr [rbp - 4], edi
mov dword ptr [rbp - 8], esi
mov eax, dword ptr [rbp - 4]
add eax, dword ptr [rbp - 8]
pop rbp
ret
example::main:
push rbp
mov rbp, rsp
sub rsp, 16
mov dword ptr [rbp - 4], 100
mov dword ptr [rbp - 8], 21
mov esi, dword ptr [rbp - 8]
mov edi, dword ptr [rbp - 4]
call example::add
mov dword ptr [rbp - 12], eax
; ... (println! macro expansion)
This assembly demonstrates Rust's direct compilation to machine code and how it manages the stack frame.
C#
For C#, we can use tools like ILSpy to examine the Intermediate Language (IL) code:
using System;
class Program
{
static int Add(int x, int y)
{
return x + y;
}
static void Main()
{
int a = 100;
int b = 21;
int c = Add(a, b);
Console.WriteLine($"The result is: {c}");
}
}
The IL code for the Main
method might look like this:
.method private hidebysig static void Main() cil managed
{
.entrypoint
.maxstack 2
.locals init (
[0] int32 a,
[1] int32 b,
[2] int32 c
)
IL_0000: ldc.i4.s 100
IL_0002: stloc.0
IL_0003: ldc.i4.s 21
IL_0005: stloc.1
IL_0006: ldloc.0
IL_0007: ldloc.1
IL_0008: call int32 Program::Add(int32, int32)
IL_000d: stloc.2
IL_000e: ldstr "The result is: {0}"
IL_0013: ldloc.2
IL_0014: box [System.Runtime]System.Int32
IL_0019: call void [System.Console]System.Console::WriteLine(string, object)
IL_001e: ret
}
This IL code shows how C# manages local variables, performs method calls, and interacts with the CLR.
These code examples and their corresponding low-level representations provide insight into how each language manages memory, performs operations, and executes at a lower level. They illustrate the different approaches to compilation and execution discussed earlier in the article.