JIT, AOT Compilations. HAL. Assembler.
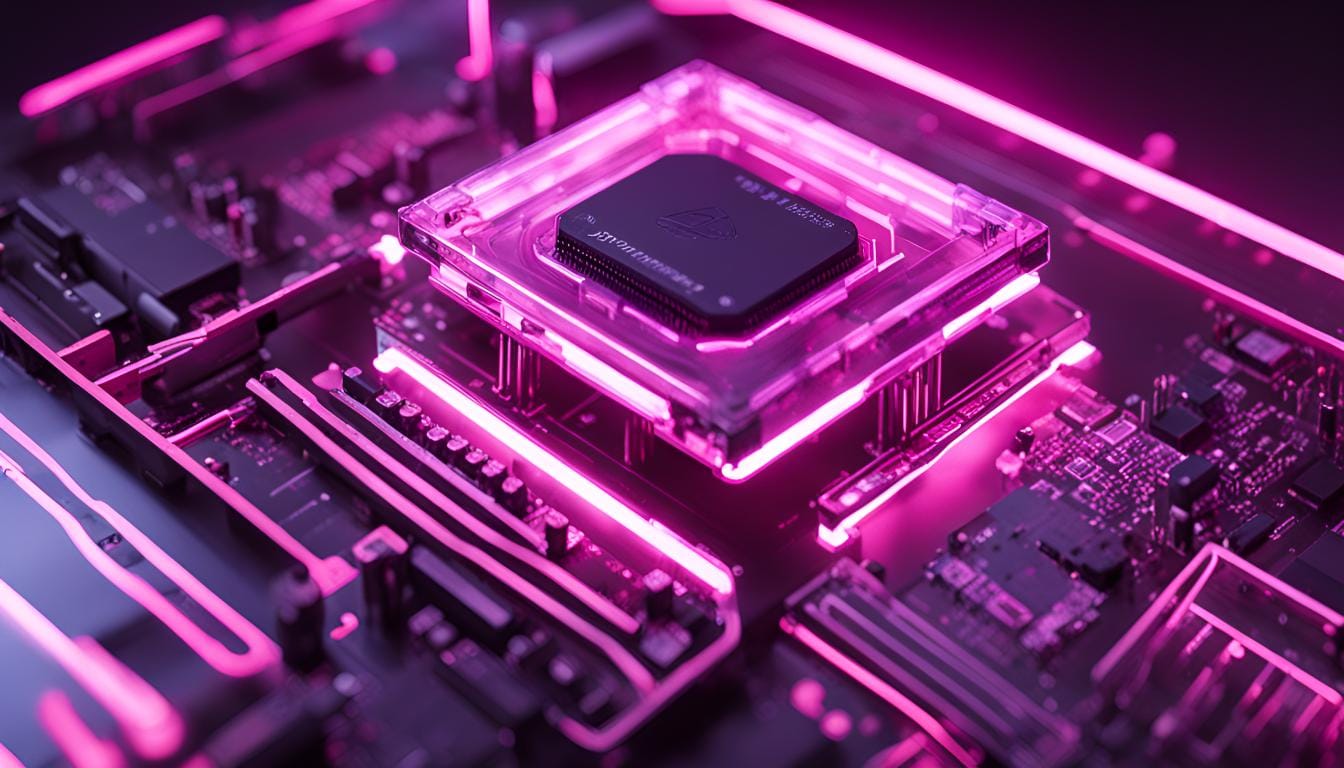
JIT-Compiled Programming Languages
Just-In-Time (JIT) compilation dynamically compiles code during runtime, allowing for optimization based on real-time execution. Here are some languages that utilize JIT compilation:
- Java: Utilizes the Java Virtual Machine (JVM) with the HotSpot JIT compiler to enhance performance.
- C#: Implements JIT through the .NET Common Language Runtime (CLR).
- JavaScript: Uses engines such as V8 (Chrome), SpiderMonkey (Firefox), and JavaScriptCore (Safari) for JIT compilation.
- Python: The PyPy and Numba implementations feature JIT compilation.
- Ruby: JRuby and TruffleRuby employ JIT compilation to improve runtime efficiency.
- Lua: LuaJIT offers high-performance JIT compilation for the Lua language.
- Erlang: The BEAM virtual machine supports JIT for efficient Erlang execution.
- PHP: Facebook’s HHVM (HipHop Virtual Machine) brings JIT compilation to PHP.
- R: Implementations such as Renjin and FastR use JIT to optimize R code.
Additional Insights on JIT Compilation
Recent research focuses on adaptive JIT compilation, where the runtime compiler can optimize hot paths in the code more aggressively. Google’s V8 engine for JavaScript, for instance, introduced TurboFan, which improves performance for dynamic languages by profiling execution paths. Similarly, for Python, PyPy continues to optimize its JIT techniques, especially in the fields of numerical computing and data analysis, where runtime performance is critical.
Ahead-Of-Time (AOT) Compiled Languages
Ahead-Of-Time (AOT) compilation converts source code into machine code prior to execution, which enhances performance by reducing the overhead associated with runtime compilation. Examples include:
- C: Compiled into machine code via compilers like GCC or Clang.
- C++: Similarly, C++ code is compiled into machine instructions.
- Rust: Uses rustc to compile directly to machine code.
- Go: Compiles into machine code using the Go compiler.
Interpreted Languages
These languages are interpreted at runtime, typically converting high-level code into an intermediate form, such as bytecode, and executing it:
- Python: CPython interprets Python code.
- Ruby: MRI (Matz’s Ruby Interpreter) handles Ruby code interpretation.
- Perl: The Perl interpreter directly runs Perl scripts.
- PHP: Zend Engine powers PHP interpretation.
Languages with Both AOT and JIT Compilation
Certain programming languages combine AOT and JIT compilation to balance performance and flexibility:
- Kotlin: Compiles to JVM bytecode and can leverage JIT within the JVM.
- Scala: Also compiles to JVM bytecode and benefits from JIT during execution.
- Clojure: Uses JVM bytecode compilation with JIT optimizations.
New Research in AOT and JIT Combination
The hybrid use of AOT and JIT has led to improvements in mobile and cloud environments. Google’s Android Runtime (ART) employs AOT for app installation, while switching to JIT for performance-sensitive runtime adaptations. This model reduces app launch times while optimizing performance dynamically.
Transpiling Languages
Transpilation involves converting code from one high-level language to another, often for execution in different environments, such as browsers. Examples include:
- TypeScript: Transpiles to JavaScript for browser or server-side execution.
- CoffeeScript: Converts to JavaScript.
- Dart: Can transpile to JavaScript to run in web browsers.
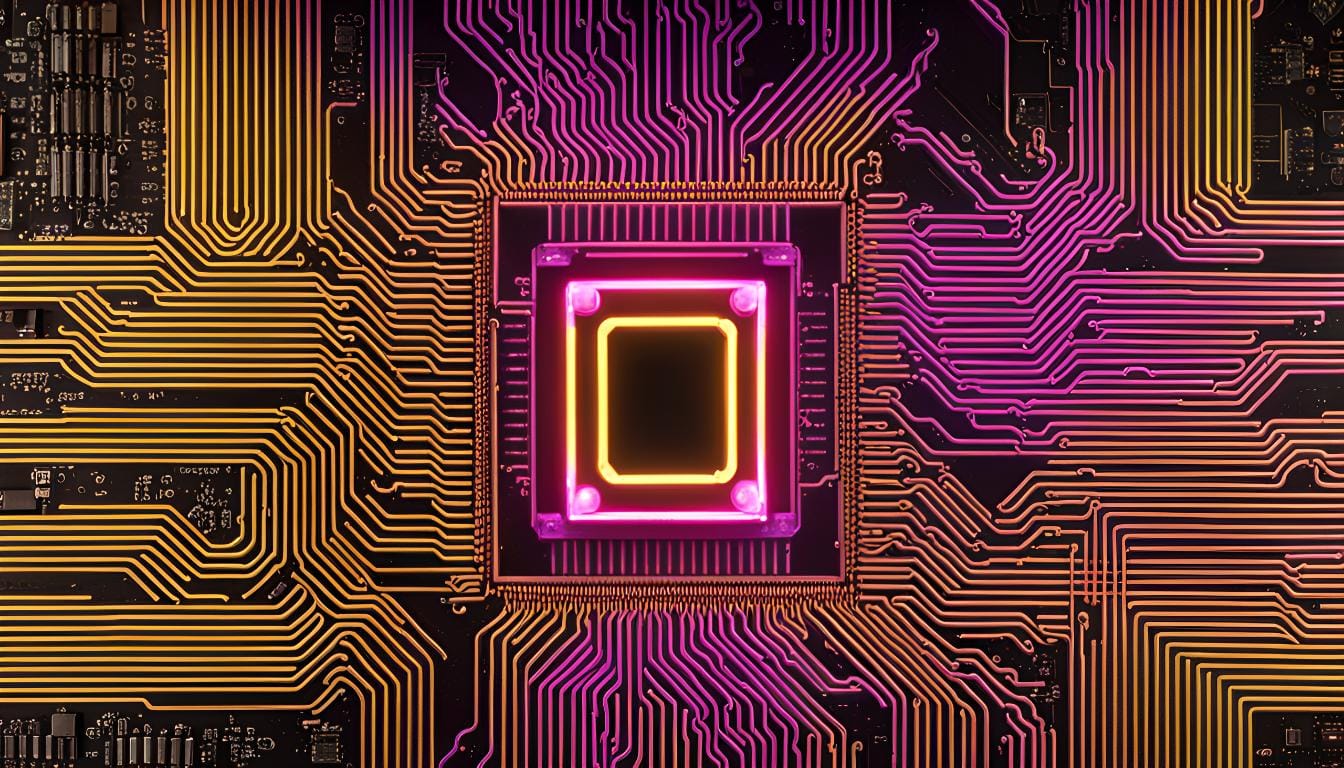
Compiler Types and Architectures
Compilers convert high-level source code into machine code, enabling efficient program execution. Here are some common compiler types:
1. Ahead-Of-Time (AOT) Compilers
An AOT compiler converts code directly into machine code before execution. GCC (GNU Compiler Collection) is a prominent example, widely used for languages like C, C++, and Objective-C.
Example: C Code Compilation
cCopy code#include <stdio.h>
{
int main() printf("Hello, World!\n"
); return 0
;
}
The gcc
compiler will compile this C code into machine code for execution on a specific platform.
2. Just-In-Time (JIT) Compilers
JIT compilers compile code dynamically during runtime, optimizing performance based on real-time conditions. For example, the HotSpot JVM for Java employs JIT to optimize the execution of frequently called code paths.
Example: Java Code
javaCopy codepublic class HelloWorld
{ public static void main(String[] args)
{ System.out.println("Hello, World!"
);
}
}
3. Interpreted Languages and Their Compilers
Interpreted languages like Python and Ruby rely on interpreters to execute code line by line. For example, CPythoninterprets Python code into bytecode and then executes it.
Example: Python Code
pythonCopy codeprint("Hello, World!"
)
4. Transpilers (Source-to-Source Compilers)
Transpilers convert code from one language to another. A common example is the TypeScript Compiler (tsc
), which converts TypeScript code into JavaScript.
Example: TypeScript to JavaScript
typescriptCopy codelet message: string = "Hello, World!"
;console.log
(message);
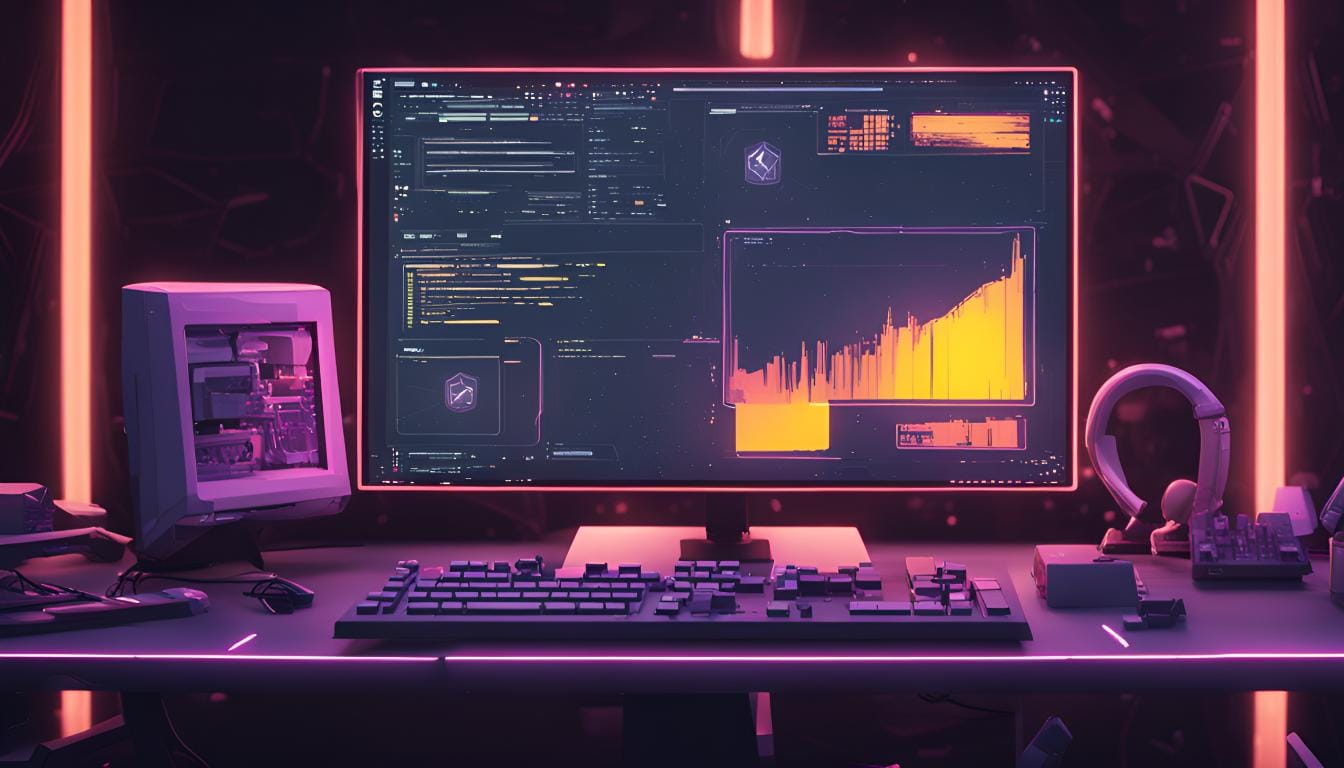
Hardware Abstraction Layer (HAL) and Kernel Architecture
Introduction to HAL
The Hardware Abstraction Layer (HAL) acts as an intermediary between hardware components and higher-level software, such as operating systems. HAL simplifies development by abstracting hardware specifics, providing a standard interface for different types of hardware.
Role of HAL in Modern Systems
HAL is particularly useful in systems where multiple hardware configurations need to be supported, such as:
- Windows: Uses HAL to abstract hardware for drivers and operating system functions.
- Linux: HAL facilitates hardware compatibility across multiple architectures.
- macOS: Implements HAL for managing devices and peripherals efficiently.
Example HAL Implementation in C
cCopy code#include <stdio.h>
{
void hal_init_device() // Initialize device
);
printf("Device initialized.\n"
}int main()
{
hal_init_device(); return 0
;
}
Assembler and Its Role in System Programming
Assembler is a low-level programming language that interacts directly with a computer’s hardware by translating instructions into machine code. It is used where performance is critical, offering maximum control over hardware.
Example Assembler Code (x86)
asmCopy codesection .data
msg db 'Hello World!', 0
section .text
global _start
_start:
mov eax, 4 ; sys_write
mov ebx, 1 ; file descriptor
mov ecx, msg ; message
mov edx, 13 ; message length
int 0x80 ; system call
mov eax, 1 ; sys_exit
xor ebx, ebx ; return 0
int 0x80 ; system call
Advantages of Using Assembler
- Direct Hardware Access: Assembler provides control over hardware resources like memory and CPU registers.
- Performance Optimization: Developers can optimize instructions for a specific processor architecture.
- Minimal Overhead: Unlike high-level languages, assembler does not introduce additional abstraction, reducing runtime overhead.
Performance Comparison: HAL vs. Assembler
Assembler allows for direct manipulation of hardware, leading to superior performance but with added complexity. In contrast, HAL offers easier development and hardware abstraction, though at the cost of some performance due to the overhead introduced by layers of abstraction.
Example: Assembler vs. HAL for Simple Addition
Assembler (x86) Example:
asmCopy codesection .data
num1 db 10
num2 db 20
result db 0
section .text
global _start
_start:
mov al, [num1]
add al, [num2]
mov [result], al
; Exit
mov eax, 1
xor ebx, ebx
int 0x80
HAL (C) Example:
cCopy code#include <stdio.h>
{
int main() int num1 = 10
; int num2 = 20
; int
result = num1 + num2; printf("Result: %d\n"
, result); return 0
;
}
Performance Impact
Assembler generally provides faster execution because it operates directly with hardware instructions. However, HAL’s higher level of abstraction simplifies the development process, allowing for easier portability and maintainability across different platforms.
Interaction of HAL, Kernels, and Compilers
The interaction between compilers, kernels, and HAL is essential in modern system programming. Compilers convert high-level source code into machine code that can be executed by the processor, while the kernel manages system resources such as memory, I/O devices, and CPU time.
Entry Point for Program Execution
In most systems, the entry point for program execution on a processor is a function like _start
. This function is usually auto-generated by the compiler and linker and serves as the initial step in running the program.
Conclusion
Assembler provides direct hardware control and is ideal for performance-critical applications, while HAL simplifies development by abstracting hardware specifics. In the modern software landscape, systems often balance both approaches—using HAL for portability and assembler for optimization in performance-sensitive areas.
By understanding the interaction between these components—HAL, assembler, compilers, and kernels—developers can create efficient and portable software that leverages the strengths of each technology.