V8 and libuv: The Core of Node.js
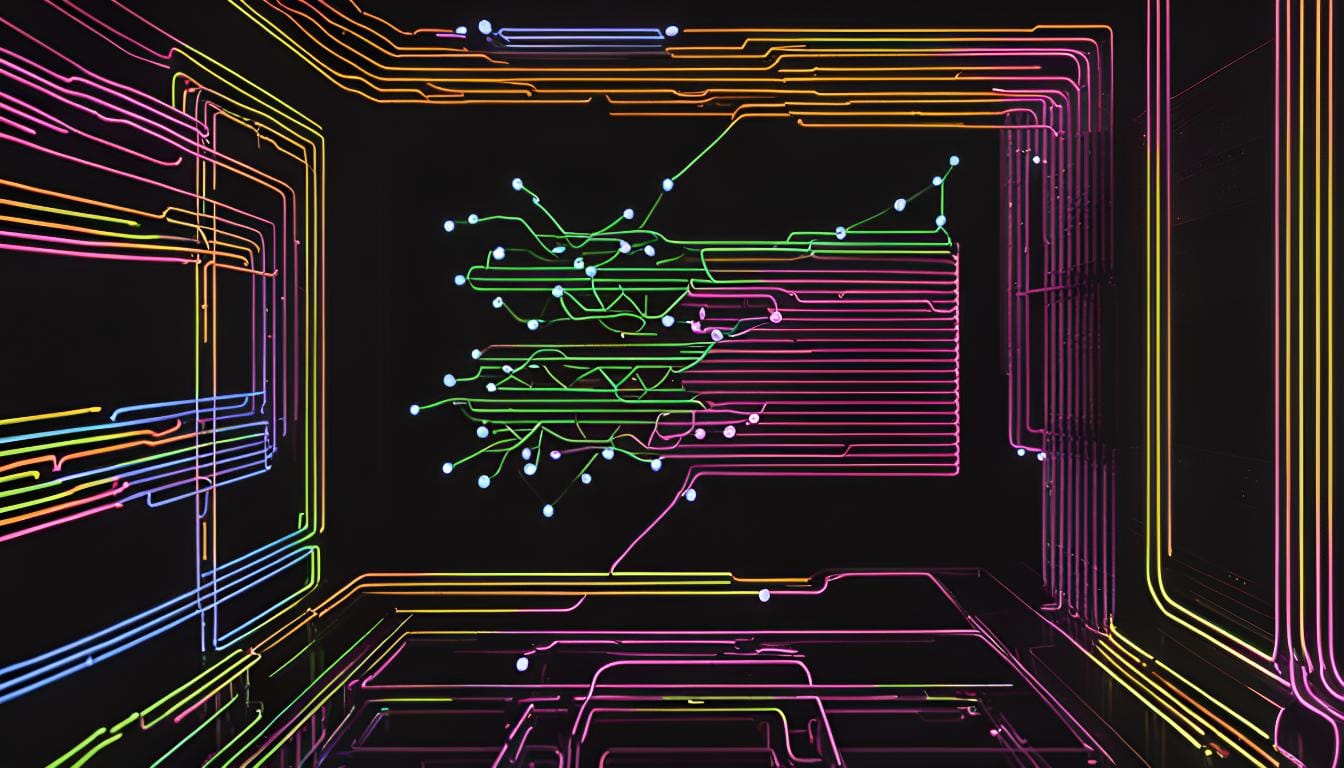
Introduction to V8
V8 is an open-source JavaScript engine developed by Google. Written in C++, it's used in Google Chrome and Node.js to execute JavaScript code. V8's primary function is to compile JavaScript into machine code, enabling extremely fast execution.
Key Components of V8
- Ignition: A bytecode interpreter that converts JavaScript code into bytecode for execution.
- TurboFan: An optimizing compiler that improves code performance by analyzing and optimizing frequently executed parts of the code.
- Orinoco: V8's garbage collection system, managing memory and preventing memory leaks.
- Runtime: A set of built-in functions and objects available in JavaScript, such as array methods and string operations.
How V8 Works
V8 first uses Ignition to interpret JavaScript code and execute it as bytecode. TurboFan then analyzes the code execution and optimizes "hot" functions by compiling them into highly efficient machine code. Orinoco manages memory throughout this process, while the Runtime provides necessary functions and objects for JavaScript execution.
Introduction to libuv
Libuv is a C library that provides asynchronous I/O operations in Node.js. It supports a non-blocking I/O model, allowing Node.js to handle multiple operations simultaneously without blocking the main thread. Libuv implements crucial features such as the event loop and thread pool.
The Synergy of V8 and libuv in Node.js
In Node.js, V8 and libuv work together to ensure efficient, asynchronous execution of JavaScript code:
- Code Execution: V8 compiles and executes JavaScript code. When the code calls an asynchronous operation (e.g., file reading or network request), V8 delegates this task to libuv.
- Event Loop: Libuv manages the event loop, which tracks the completion of asynchronous operations. When an operation is completed, libuv adds the corresponding event to the event queue.
- Event Processing: When V8 is ready to process new events, it retrieves them from the event queue and invokes the appropriate callbacks.
- Thread Pool: For heavier operations like file I/O, libuv uses a thread pool. This allows such operations to be performed in parallel without blocking the main thread.
The Single Event Loop Model
Node.js utilizes a single event loop managed by libuv. This design allows for efficient handling of asynchronous operations:
- Libuv implements the main event loop, managing all asynchronous operations.
- V8 interacts with libuv's event loop rather than having its own.
- When JavaScript code initiates an asynchronous operation, V8 hands it off to libuv and continues executing other tasks.
- Upon completion of an asynchronous operation, libuv notifies V8, which then executes the corresponding callback.
Mathematical Operations in V8
V8 performs mathematical operations using C++. As V8 is written in C++, it leverages the language's capabilities for various operations, including complex mathematical calculations. This approach allows V8 to efficiently compile and execute JavaScript code:
- Compilation: JavaScript code containing mathematical operations is compiled into machine code using Just-In-Time (JIT) compilation.
- Execution: The compiled machine code is executed directly by the processor, utilizing optimized algorithms and data structures implemented in C++.
For instance, when you write Math.sqrt(16)
in JavaScript, V8 compiles this call into machine code, which is then executed by the processor. Internally, V8 uses C++ functions to perform such operations, ensuring high performance.
Conclusion
The combination of V8 and libuv forms the core of Node.js, enabling it to be both efficient and scalable. V8's powerful JavaScript execution capabilities, coupled with libuv's asynchronous I/O handling, allow Node.js to process multiple asynchronous operations simultaneously, making it an excellent choice for building high-performance, scalable applications.